- Removed 7zip unpack option.
This is now a built-in SABnzbd function
Hi folks,
Here is my Python3 script SabToSyno.py.
Some of those functions were already present in my retired [Synology NAS] Fixed non-ASCII chars and more...(2020/09/11) script.
With SABnzbd 3.x running with Python3 my script is not anymore useful. The default usage of the unicode UTF-8 in Python3 resolved the non-ASCII characters issues. However in this old script, some useful optional parameters to move downloaded file(s) and to add them in the Synology DLNA were present.
Thus, the goal of this post-processing script is to automatically add the downloaded multimedia file in the Synology DLNA server.
To do so, the script is executing the Synology command line /usr/syno/bin/synoindex.
To install the script, you must be connected to your Synology NAS through a Telnet/SSH session.
All the instructions below are for the SynoCommunity SABnzbd version:
- You must open the SSH connection with your NAS using your "admin" login credentials.
- You must switch in "root" mode by executing the command "sudo":
Code: Select all
admin@NAS:> sudo -i
- Go in the SABnzbd scripts folder, then create/copy the script here (name can be SabToSyno.py).
Code: Select all
root@NAS:> cd /var/packages/sabnzbd/target/var/scripts/
- Be sure that the file owner is correct and the script can be executed.
If not, then you can change the owner and permissions with those commands:
Code: Select all
root@NAS:> ls -l total 372 [...] -rwxr-xr-x 1 sc-sabnzbd sabnzbd 6520 Sep 19 16:30 SabToSyno.py [...]
Code: Select all
root@NAS:> chown sc-sabnzbd:sabnzbd SabToSyno.py root@NAS:> chmod 755 SabToSyno.py
- Optionally, setup the option to move the downloaded NZB to a dedicated destination.
Code: Select all
MoveToThisFolder = ''
[...]
MoveMergeSubFolder = True
If the option is filed-up with a path (e.g. MoveMergeSubFolder = '/volume1/MY_DOWLOAD/MY_VIDEO/'), the downloaded NZB will be moved to this destination folder. Then, after the move operation, the multimedia files will be added in the Syno DLNA from their new destination folder.
Important: the specified path is the same one as visible through a Telnet/SSH session. In others words, it is the true "Linux" path (case sensitive).
For example:
Code: Select all
MoveToThisFolder = '/volume1/video/Movies'
If MoveMergeSubFolder = False, then it is equivalent to unix command:
Code: Select all
mv -rf srcFolder destFolder
If MoveMergeSubFolder = True, then it is equivalent to unix command:
Code: Select all
cp -rf srcFolder/* destFolder/
rm -rf srcFolder
The script must be copied in the SABnzbd scripts folder as explained below.
The name of the script can be SabToSyno.py
Here is the code:
Code: Select all
#!/usr/local/python3
#
# Create by LapinFou.
# https://forums.sabnzbd.org/viewtopic.php?f=9&p=122338
#
# date | version | comment
#---------------------------------------
# 2020-09-13 | 1.0 | Initial version
# 2020-09-19 | 1.1 | Code clean-up
# 2020-09-25 | 1.2 | Removed 7zip recursive unpack option
#
# get library modules
import sys
import os
import subprocess
import shutil
scriptVersionIs = 1.2
# If empty, then no move
# Format must be synology full path (case sensitive). For ex: /volume1/video/News
MoveToThisFolder = ''
# If MoveMergeSubFolder = False, then equivalent to unix command:
# mv -rf srcFolder destFolder
# In case of conflict between an already existing sub-folder in the destination folder:
# the destination sub-folder will be replaced with source sub-folder
#
# If MoveMergeSubFolder = True, then equivalent to unix command:
# cp -rf srcFolder/* destFolder/
# rm -rf srcFolder
# In case of conflict between an already existing sub-folder in the destination folder:
# the destination sub-folder will be merged with source sub-folder (kind of incremental)
MoveMergeSubFolder = True
########################
# ----- Functions ---- #
########################
# Get information from SABnzbd
try:
(scriptname,directory,orgnzbname,jobname,reportnumber,category,group,postprocstatus,url) = sys.argv
except:
print("No commandline parameters found")
sys.exit(1)
# add folder in the Syno index database (DLNA server)
def addToSynoIndex(DirName):
print("Adding folder in the DLNA server")
synoindex_cmd = ['/usr/syno/bin/synoindex', '-A', DirName]
try:
p = subprocess.Popen(synoindex_cmd, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
out, err = p.communicate()
out = out.decode('ascii')
err = err.decode('ascii')
if (str(out) == ''):
print("synoindex result: " + DirName + " successfully added to Synology database")
else:
print("synoindex result: " + str(out))
if (str(err) != ''):
print("synoindex failed: " + str(err))
except OSError as e:
print("Unable to run synoindex: "+str(e))
return
########################
# --- Main Program --- #
########################
print("Running SabToSyno Python3 script (v%s)" %scriptVersionIs)
print("")
# Change current directory to SABnzbd "complete" directory
os.chdir(directory)
# display directory of the SABnzbd job
currentFolder = os.getcwd()
print("Current folder is " + currentFolder)
# Move current folder to an another destination if the option has been configured
if (MoveToThisFolder != ''):
print("")
print(100*'-')
print("Moving folder:")
print(currentFolder)
print("to:")
print(MoveToThisFolder)
# Check if destination folder does exist and can be written
# If destination doesn't exist, it will be created
if (os.access(MoveToThisFolder, os.F_OK) == False):
os.makedirs(MoveToThisFolder)
os.chmod(MoveToThisFolder, 0o777)
# Check write access
if (os.access(MoveToThisFolder, os.W_OK) == False):
print(100*'#')
print("File(s)/Folder(s) can not be move in %s" %(MoveToThisFolder))
print("Please, check Unix permissions")
print(100*'#')
sys.exit(1)
# If MoveMergeSubFolder is True, then move all file(s)/folder(s) to destination
# then remove source folder
destFolder = os.path.join(MoveToThisFolder, os.path.split(currentFolder)[-1])
if (MoveMergeSubFolder):
print(" Info: Merge option is ON (incremental copy)")
try:
synoCopy_cmd = ['/bin/cp', '-Rpf', os.path.abspath(currentFolder), MoveToThisFolder]
p = subprocess.Popen(synoCopy_cmd, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
out, err = p.communicate()
out = out.decode('ascii')
err = err.decode('ascii')
if (str(err) != ''):
print("Copy failed: " + str(err))
sys.exit(1)
except OSError as e:
print("Unable to run cp: " + str(e))
sys.exit(1)
os.chdir(MoveToThisFolder)
shutil.rmtree(currentFolder)
# If MoveMergeSubFolder is False, remove folder with same (if exists)
# then move all file(s)/folder(s) to destination and remove source folder
else:
print(" Info: Merge option is OFF (existing data will be deleted and replaced)")
# Remove if destination already exist
if os.path.exists(destFolder):
shutil.rmtree(destFolder)
shutil.move(currentFolder, MoveToThisFolder)
else:
# If move is not enabled, the current folder will be indexed
destFolder = currentFolder
# Add multimedia files in the Syno DLNA
print("")
print(100*'-')
addToSynoIndex(destFolder)
print("")
print("Moving and indexing file(s) done!")
# Success code
sys.exit(0)
http://sab_url:8080/config/categories/
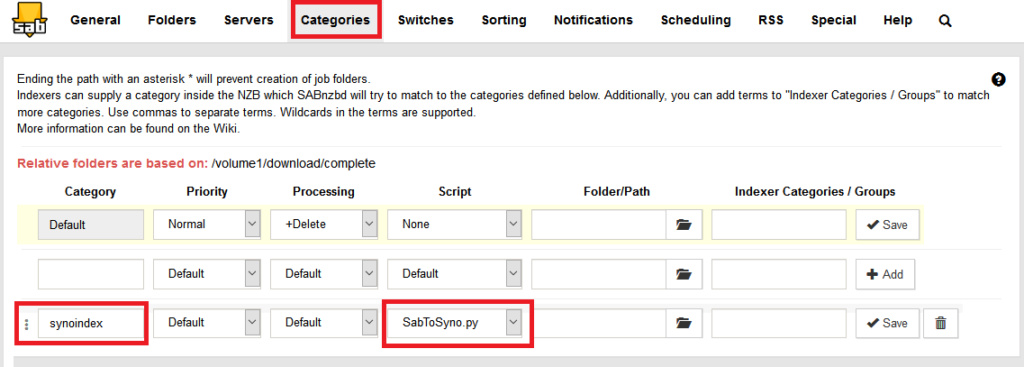
When a NZB is downloaded, select the created category:
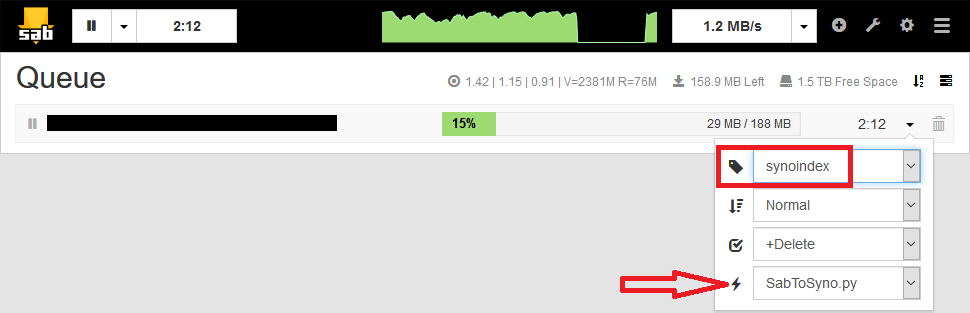